What is PowerShell Dot Sourcing?
Dot sourcing in PowerShell is a method used to execute a script or a function in the current scope rather than in a new, separate scope (which is the default behavior). This allows the variables, functions, and aliases defined in the script to persist in the current session after the script finishes execution.
Normally, when you run a script or function in PowerShell, a new scope is created, meaning that any variables or functions defined in the script are not available once the script finishes.
Dot sourcing overrides this behavior, making the contents of the script available to the caller.
To dot source a script, use a dot ( . ) followed by a space and then the path to the script or function you want to execute.
PS C:\> . C:\path\to\script.ps1 dot-sourcing a script from the current directory/path PS C:\> . .\script.ps1
Assume we have a script called example.ps1 with the following content:
# example.ps1 $greeting = "Hello, PowerShell!" function Show-Greeting { Write-Host $greeting }
Without dot sourcing the script runs, but once it’s done, neither $greeting nor the Show-Greeting function will be available in the current session.
If you try to access $greeting or run Show-Greeting, PowerShell will throw an error because they are not defined in your current scope.
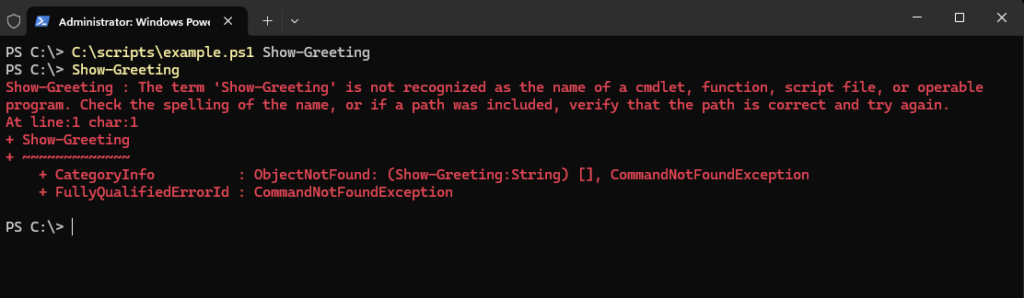
With dot sourcing the script runs, and after it completes, the $greeting variable and Show-Greeting function remain available in the current session.
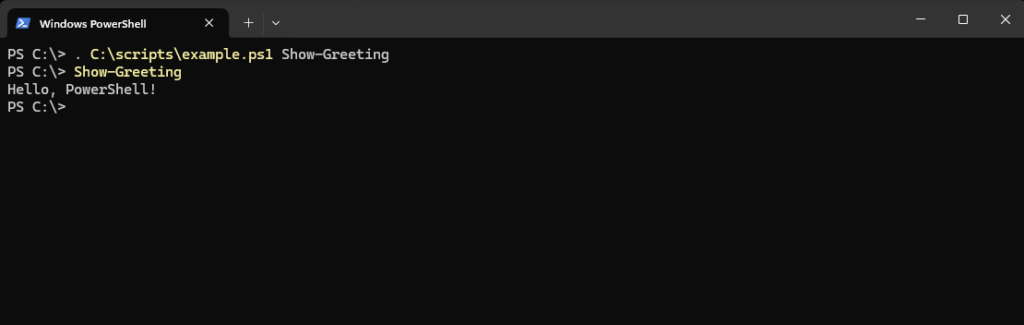
Dot sourcing the script from the current directory/path.
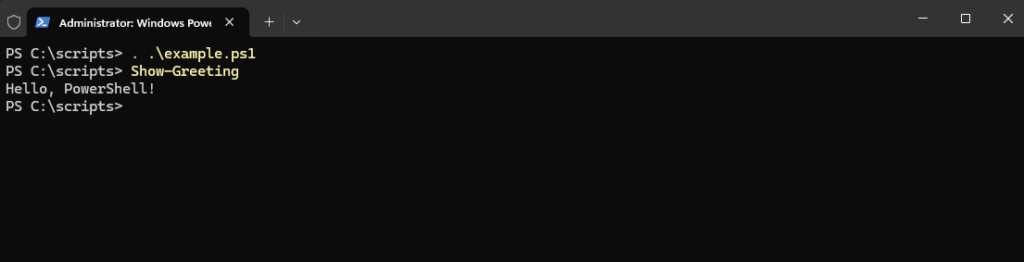
Dot sourcing is often used to load helper functions from a script into the current session without executing the entire script again each time.
PS C:\> . C:\Scripts\Utilities.ps1
Now, all the utility functions defined in Utilities.ps1 can be used in the current session.
Dot sourcing in PowerShell is a powerful feature that allows you to run a script or function while keeping the defined variables and functions available in the current session. This is useful when you want to load functions or variables without having to rerun an entire script multiple times.
Links
Script scope and dot sourcing
https://learn.microsoft.com/en-us/powershell/module/microsoft.powershell.core/about/about_scripts?view=powershell-7.4#script-scope-and-dot-sourcingDot-Sourcing Functions
https://learn.microsoft.com/en-us/powershell/scripting/learn/ps101/10-script-modules?view=powershell-7.4#dot-sourcing-functions
Tags In
Latest posts
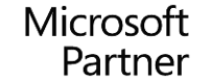