Enable Python for Apache Websites
Below I want to show how you can enable python for websites running on the apache webserver in Ubuntu.
Install Python
Python3 is by default installed on Ubuntu as required by system components.
You can nevertheless install Python3 by using the following command.
$ sudo apt install python3
# Determin the version
$ python3 -V
By default you will execute Python3 with the python3 command, by installing the packet below, you can execute Python3 also as usual with the command python.
$ sudo apt install python-is-python3
Set up Apache
$ sudo apt install libapache2-mod-python
The mod_python module supports web applications written in Python. Because the parser is embedded in the server as an Apache module, it will run much faster than traditional CGI.
Source: https://packages.debian.org/sid/libapache2-mod-python$ sudo a2enmod cgi
$ sudo a2enmod mpm_prefork
Enable Python in the Apache Virtual Host Site
<IfModule mod_ssl.c> <VirtualHost *:443> DocumentRoot /var/www/html <Directory /var/www/html/> DirectoryIndex index.py Options +ExecCGI AddHandler cgi-script .py Require all granted # PythonDebug On </Directory> </VirtualHost> </IfModule>
Explicitly using Options to permit CGI execution
You could explicitly use the Options directive, inside your main server configuration file, to specify that CGI execution was permitted in a particular directory:
<Directory "/usr/local/apache2/htdocs/somedir"> Options +ExecCGI </Directory>
The above directive tells Apache to permit the execution of CGI files. You will also need to tell the server what files are CGI files. The following AddHandler directive tells the server to treat all files with the cgi or py extension as CGI programs:
AddHandler cgi-script .cgi .py
The CGI (Common Gateway Interface) defines a way for a web server to interact with external content-generating programs, which are often referred to as CGI programs or CGI scripts. It is a simple way to put dynamic content on your web site, using whatever programming language you’re most familiar with. This document will be an introduction to setting up CGI on your Apache web server, and getting started writing CGI programs.
Source: https://httpd.apache.org/docs/2.4/howto/cgi.html
Python Test Script Hello World for Websites
#! /usr/bin/python3 a = 200 b = 350 sum = int(a) + int(b) print("Content-type:text/plain;charset=utf-8nn") print ("Hello World!") print () print ("The sum is: ", sum)
Run a Python Script in Ubuntu
You can write python scripts in classic text editors. If you want to run the script in the console, you can either use the python command to call the python script file or you can insert a so called shebang into the python script file, in order to execute the script directly without using the python command.
If you just enter the name from the script in the console, even you added before the execute rights as shown below, you can’t run the script.
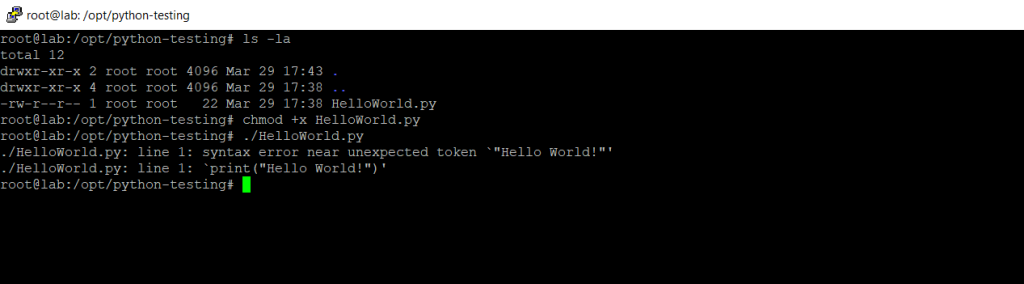
By using the python3 command in front of you can execute the python script.
$ sudo python3 scriptname.py
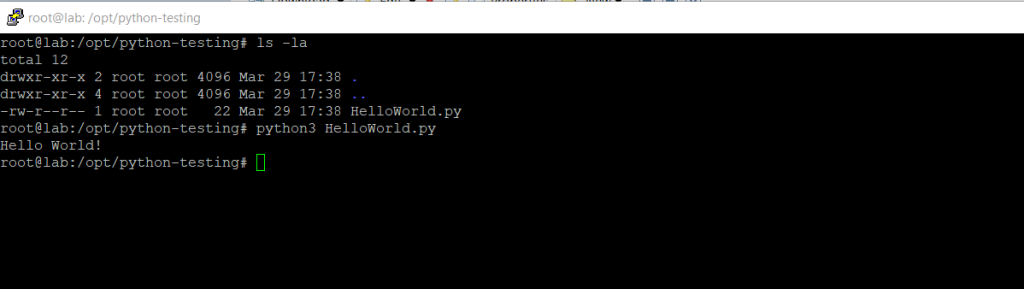
To execute the python script directly by just enter the name from the script, we first need to add the following shebang in the first line of the script.
The shebang is used to tell the system which interpreter for the execution of the script should be used.
#! /usr/bin/python3
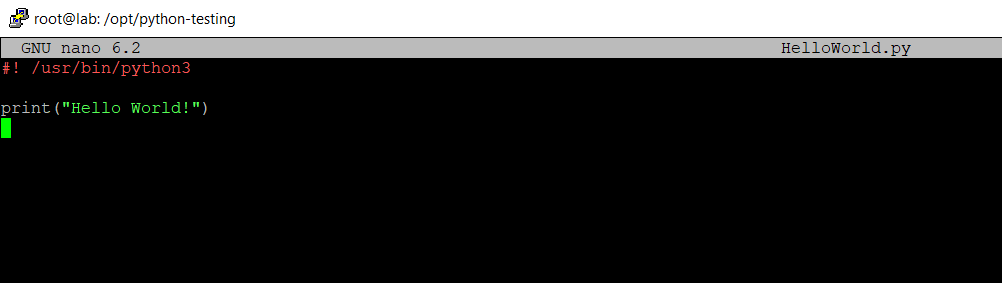
Now we can execute the script by just enter the name.
Provided the execution rights where added to the script
$ sudo chmod +x HelloWorld.py
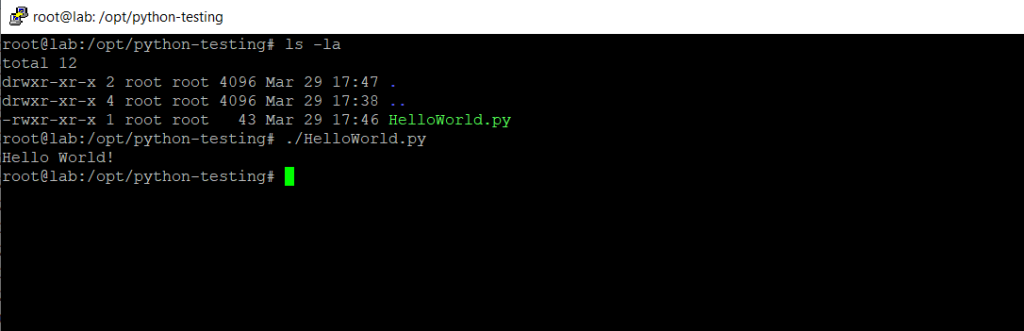
Links
How To Make a Web Application Using Flask in Python 3
https://www.digitalocean.com/community/tutorials/how-to-make-a-web-application-using-flask-in-python-3Python Tutorial
https://www.w3schools.com/python/Shebang (Unix)
https://en.wikipedia.org/wiki/Shebang_(Unix)python
https://www.python.org/Apache Tutorial: Dynamic Content with CGI
https://httpd.apache.org/docs/2.4/howto/cgi.html