Run a .NET Core C# Console App on Ubuntu
In this post we will see how we can run a .NET Core Console App on Ubuntu. Therefore I will use a little C# console app which will just create a TCP/IP socket and waiting for incoming TCP/IP connections.
Introduction
In order to run .NET Core applications on Ubuntu, we first need to install the .NET SDK or .NET Runtime on Ubuntu.
You can also publish your app as self-contained, which produces an application that includes the .NET runtime and libraries and your application and its dependencies. Users of the application can run it on a machine that doesn’t have the .NET runtime installed. We will see this further down.
Source: https://learn.microsoft.com/en-us/dotnet/core/deploying/
Install the SDK (which includes the runtime) if you want to develop .NET apps. Or, if you only need to run apps, install the Runtime. If you’re installing the Runtime, we suggest you install the ASP.NET Core Runtime as it includes both .NET and ASP.NET Core runtimes.
Use the
dotnet --list-sdks
and
dotnet --list-runtimes
commands to see which versions are installed. For more information, see How to check that .NET is already installed.
.NET is available in the Ubuntu package manager feeds, as well as the Microsoft repository. However, you should only one use or the other to install .NET. Review the Decide how to install .NET section to determine which feed you should use.
Don’t use both repositories to manage .NET. If you’ve previously installed .NET from the Ubuntu feed or the Microsoft feed, you’ll run into issues using the other feed. .NET is installed to different locations and is resolved differently for both package feeds. It’s recommended that you uninstall previously installed versions of .NET and then install with the Microsoft package repository. For more information, see How to register the Microsoft package repository.
Source: https://learn.microsoft.com/en-us/dotnet/core/install/linux-ubuntu-2210
The Microsoft package repository contains every version of .NET that is currently, or was previously, supported on Ubuntu. Starting with Ubuntu 22.04, some versions of .NET are available in the Ubuntu package feed. For more information about available versions, see the Supported distributions section.
Source: https://learn.microsoft.com/en-us/dotnet/core/install/linux-ubuntu
So I will use the Microsoft package repository and therefore first remove potentially previous installed packages by executing the following commands.
$ sudo apt remove dotnet* aspnetcore* netstandard*
$ sudo apt autoremove
So finally no runtime or SDK is installed on the system.
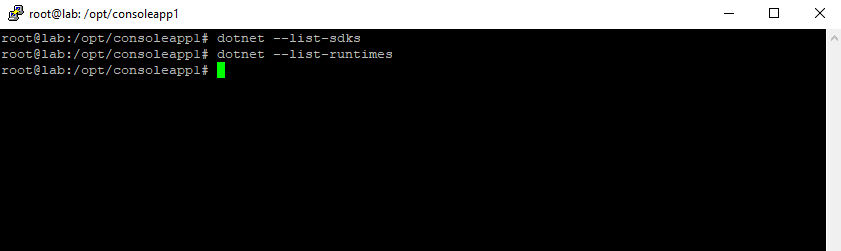
To use the Microsoft repository we first need to configure the Ubuntu package manger to ignore the .NET packages from the distribution’s repository.
Configure your package manager to ignore the .NET packages from the distribution’s repository. It’s possible that you’ve installed .NET from both repositories, so you want to choose one or the other.
Create a new file named for example 99microsoft-dotnet.pref in /etc/apt/preferences.d/ in an editor and add the settings below, which prevents packages that start with dotnet, aspnetcore, or netstandard from being sourced from the distribution’s repository.
Make sure to replace with your distribution’s package source, for example, on Ubuntu you may use archive.ubuntu.com in the US.
You can check which distribution’s package source is used on your system easily by open the /etc/apt/sources.list file.
$ nano /etc/apt/sources.list
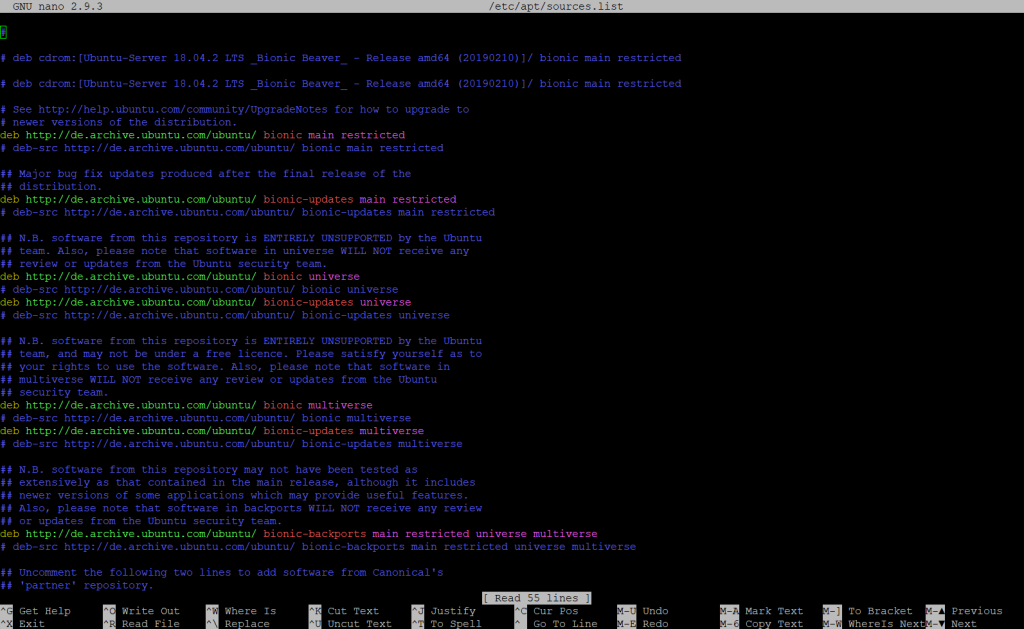
For Germany and in my case this is de.archive.ubuntu.com.
Package: dotnet* aspnet* netstandard* Pin: origin "de.archive.ubuntu.com" Pin-Priority: -10
In case you have problems by using the Microsoft repository, you can of course also try the distribution’s package source. If so, first remove the previously installed Microsoft packages as shown to the beginning, then simply change the origin URI above from de.archive.ubuntu.com into packages.microsoft.com and finally re-install the runtime or SDK.
apt_preferences – Preference control file for APT
https://manpages.ubuntu.com/manpages/bionic/en/man5/apt_preferences.5.html
Installing with APT can be done with a few commands. Before you install .NET, run the following commands to add the Microsoft package signing key to your list of trusted keys and add the package repository.
# Get Ubuntu version declare repo_version=$(if command -v lsb_release &> /dev/null; then lsb_release -r -s; else grep -oP '(?<=^VERSION_ID=).+' /etc/os-release | tr -d '"'; fi) # Download Microsoft signing key and repository wget https://packages.microsoft.com/config/ubuntu/$repo_version/packages-microsoft-prod.deb -O packages-microsoft-prod.deb # Install Microsoft signing key and repository sudo dpkg -i packages-microsoft-prod.deb # Clean up rm packages-microsoft-prod.deb # Update packages sudo apt update
After you’ve registered the Microsoft package repository, or if your version of Ubuntu’s default feed supports the .NET package, you can install .NET through the package manager with the apt install command. Replace with the name of the .NET package you want to install. For example, to install .NET SDK 7.0, use the command apt install dotnet-sdk-7.0. Currently supported .NET packages you will find in the link below.
Install .NET
https://learn.microsoft.com/en-us/dotnet/core/install/linux-ubuntu#install-net
Install the .NET Runtime on Ubuntu
As I don’t want to develop on Ubuntu, I will just install the .NET Core 7.0 runtime by executing the following command.
$ sudo apt install aspnetcore-runtime-7.0
Below you can see that packages from the Microsoft repository will be used to install the .NET runtime as preferred.
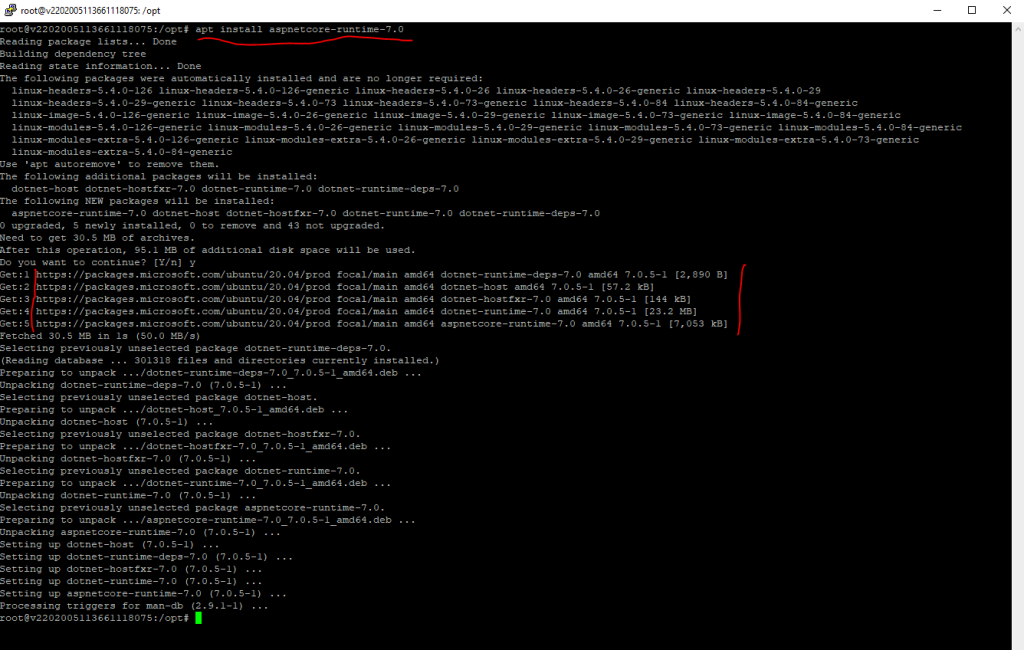
Install the .NET SDK on Ubuntu
To install the .NET SDK we can use the following command.
$ sudo apt install dotnet-sdk-7.0
Also for the SDK, packages from the Microsoft repository will be used as preferred.
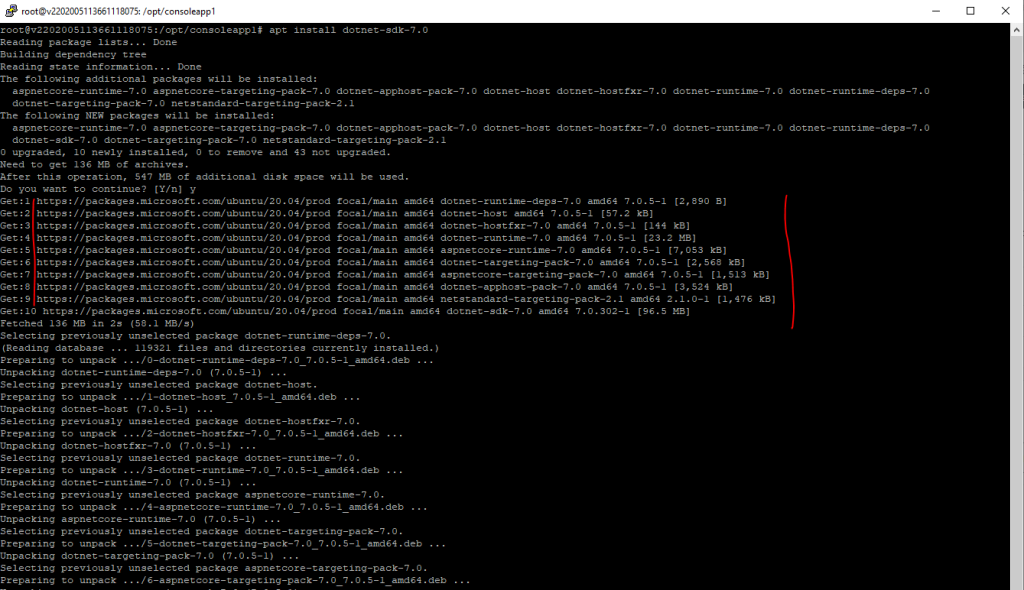
Publish .NET Console App
Now as we have prepared our Ubuntu machine to be able to run .NET Core applications, I will publish my little C# console app.
using System.Net.Sockets; using System.Net; using System; // See https://aka.ms/new-console-template for more information //Console.WriteLine("Hello, World!"); class Server { public static void Main() { TcpListener server = new TcpListener(IPAddress.Parse("127.0.0.1"), 8080); server.Start(); Console.WriteLine("Server has started on 127.0.0.1:8080.{0}Waiting for a connection…", Environment.NewLine); TcpClient client = server.AcceptTcpClient(); Console.WriteLine("A client connected."); } }
Tools –> Command Line –> Developer Command Prompt
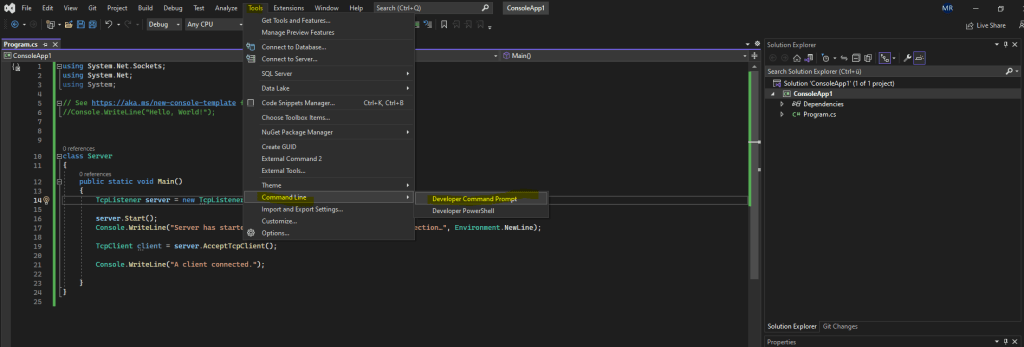
dotnet publish -r linux-x64 --self-contained true
Publishing your app as self-contained, produces an application that includes the .NET runtime, libraries and your application and its dependencies. Users of the application can run it on a machine that doesn’t have the .NET runtime installed.
dotnet publish -r linux-x64 --self-contained false
Publishing your app as framework-dependent, produces an application that includes only your application itself and its dependencies. Users of the application have to separately install the .NET runtime.
Source: https://learn.microsoft.com/en-us/dotnet/core/deploying/
By the way when trying to run a .NET app which depends on the .NET framework on Ubuntu, where neither the .NET runtime nor the SDK is installed, we will run into the following error.
You must install .NET to run this application.
.NET location: Not found
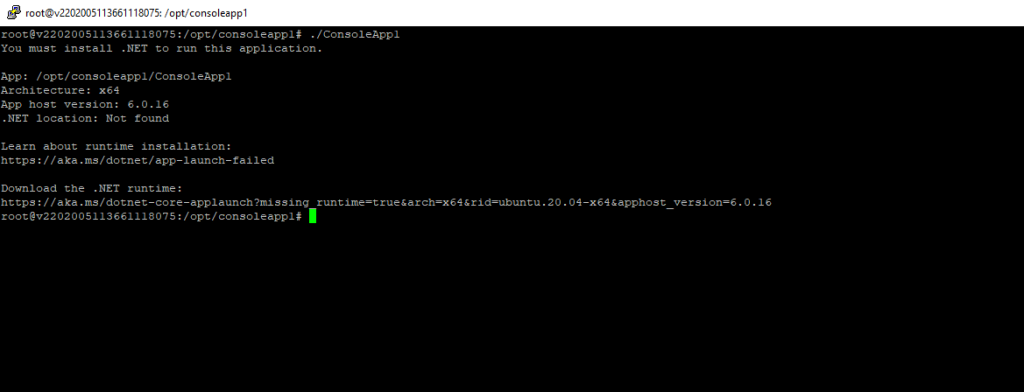
Below I will publish the app as self-contained.
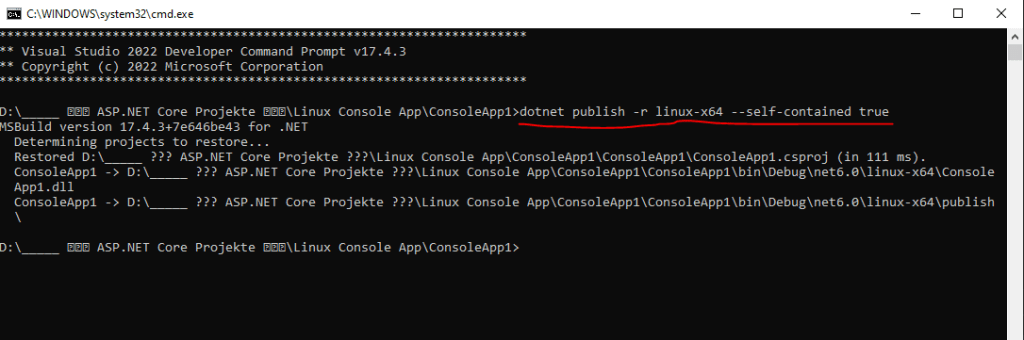
Grant execution permissions for the ConsoleApp1 application by executing the following command.
$ sudo chmod u+x ConsoleApp1
This will grant execution permissions for the owner of the file by using the u flag for user. For all users you can use the a flag or just the following syntax:
$ sudo chmod +x ConsoleApp1
- u stands for user.
- g stands for group.
- o stands for others.
- a stands for all.
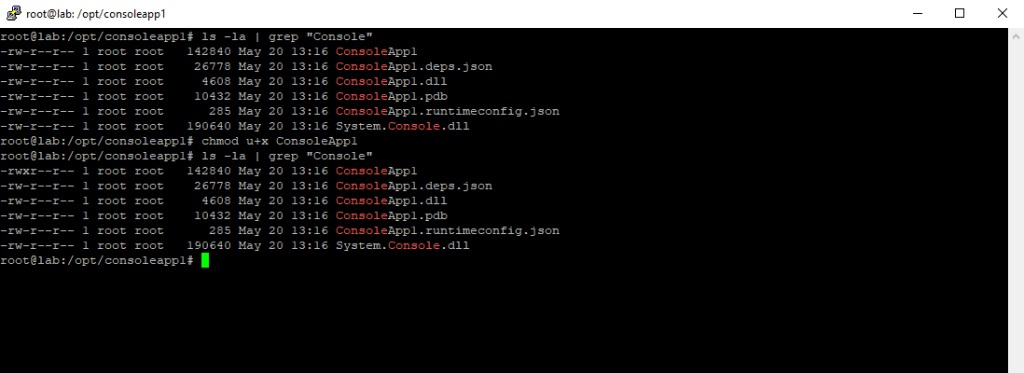
Starting the app, looks good!
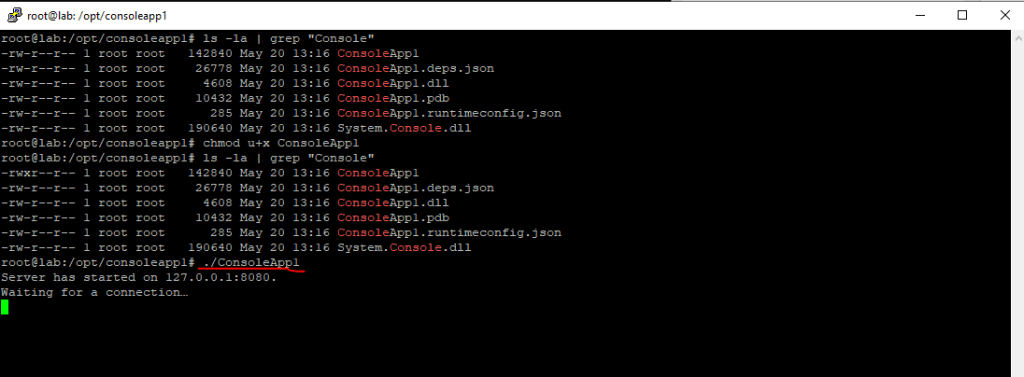
Links
Install .NET on Windows, Linux, and macOS
https://learn.microsoft.com/en-us/dotnet/core/install/Install the .NET SDK or the .NET Runtime on Ubuntu
https://learn.microsoft.com/en-us/dotnet/core/install/linux-ubuntuInstalling .NET 6 on Ubuntu 22.04 (Jammy)
https://github.com/dotnet/core/issues/7699Troubleshoot .NET errors related to missing files on Linux
https://learn.microsoft.com/en-us/dotnet/core/install/linux-package-mixup?pivots=os-linux-redhat