Blazor Server – Basics Part 2
As mentioned in Part 1, in this post I will show how to create a new blazor server web app and some further basic information.
To build your first web app with Blazor server I would also recommend to read the following intro from Microsoft which I was also using first.
Blazor Tutorial – Build your first Blazor app
https://dotnet.microsoft.com/en-us/learn/aspnet/blazor-tutorial/intro
Create a new Blazor server web app
I will use here Visual Studio 2022 and filter for templates about C#, on Windows and for the Web. You can also see here the template for a Blazor WebAssembly App.
I will choose the Blazor Server App template.
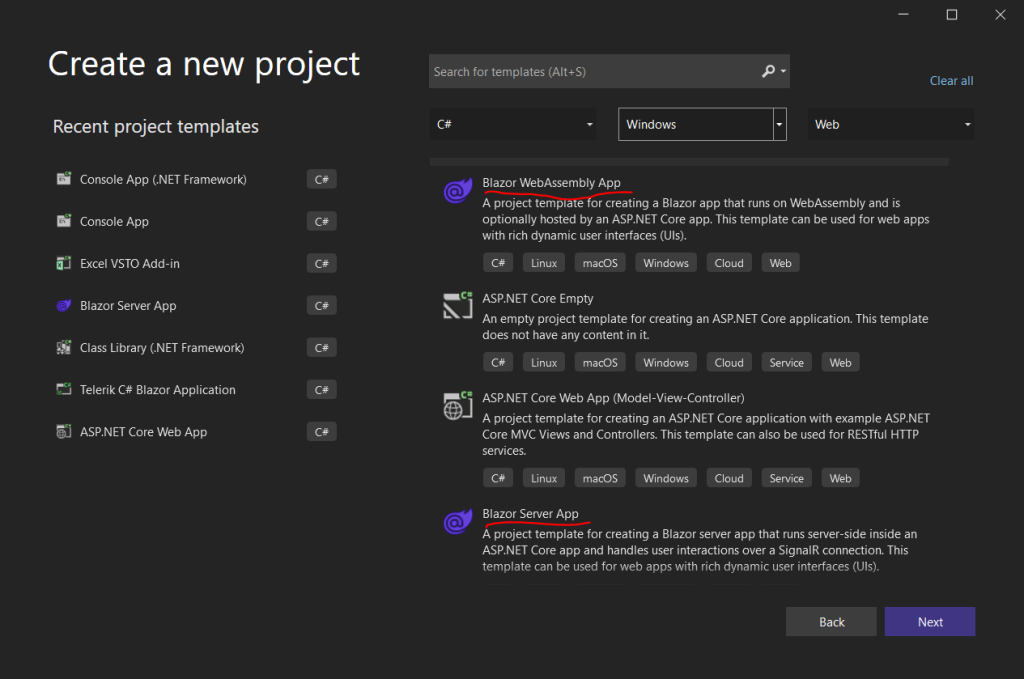
Blazor Server was included as part of .NET 5.0.
As of today, .NET 7.0 is the latest version, I will use it for the new blazor server web app here.
To get the latest .NET version you can run the Visual Studio installer.
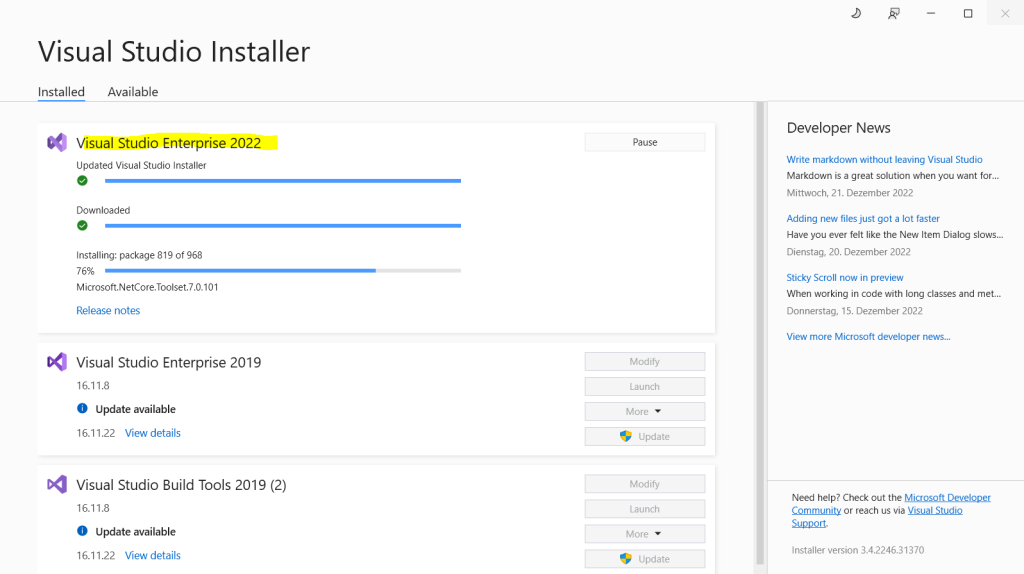
Select the .NET version you want to use, as mentioned, I will use .NET 7.0 for the new web app.
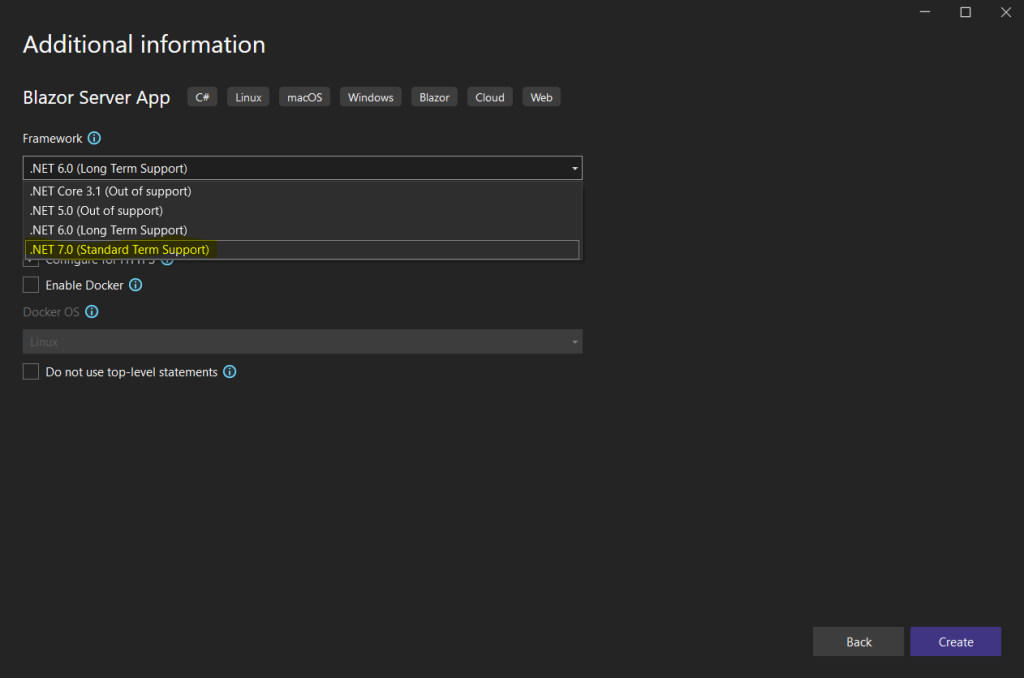
As I want to authenticate users in my web app by using the on-premise Active Directory, I will select here Windows, for Azure AD you can select Microsoft identity platform.
Finally click on Create.
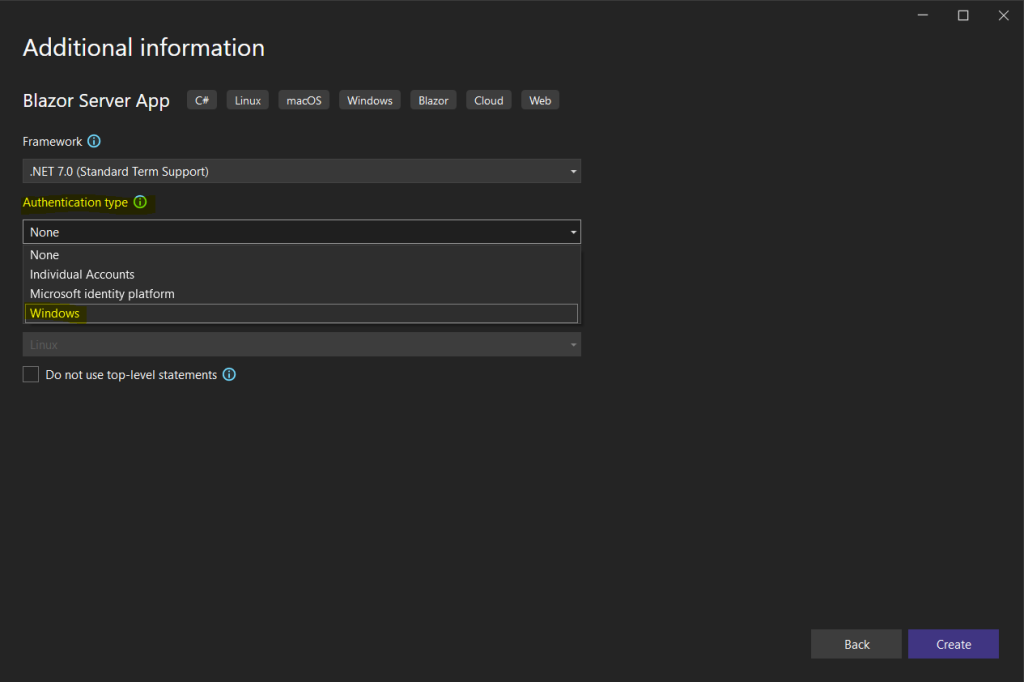
Finally our blazor server template was created with a bunch of files and folders you can see below red marked. I will explain the separate files and folders below.
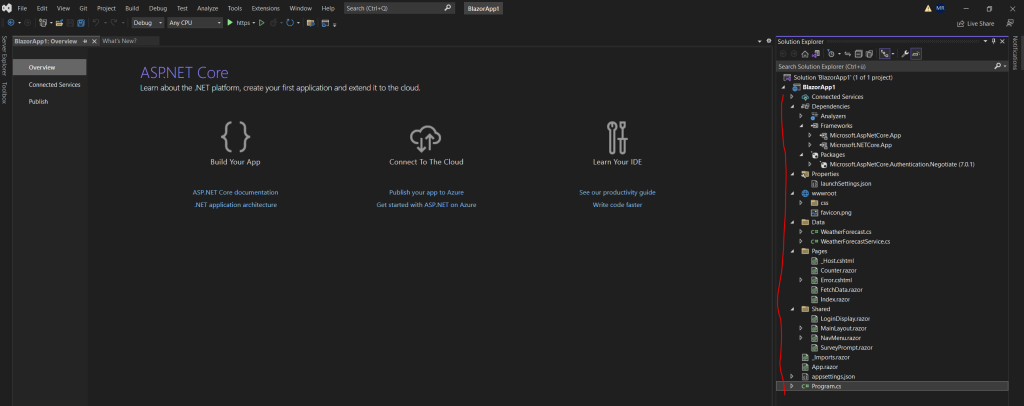
Blazor project structure
ASP.NET Core Blazor project structure
https://learn.microsoft.com/en-us/aspnet/core/blazor/project-structure?view=aspnetcore-7.0
Under Dependencies you will find as used to several assemblies used by the project. Inside the Properties folder you will find a launchSettings.json file which will defines different profile settings for the local development environment.
The wwwroot folder will include all static files the web app needs like css files, JavaScript files and images.
The Data folder will include the C# classes used for the web app, by default two sample classes are included by the template named WeatherForecast.cs and WeatherForecastService.cs.
The Pages folder will include your web app pages which in Blazor terminology called routable compontents/pages. In Blazor server you didn’t really have separate web pages as it is a single-page application (SPA).
A single-page application (SPA) is a web application or website that interacts with the user by dynamically rewriting the current web page with new data from the web server, instead of the default method of a web browser loading entire new pages. The goal is faster transitions that make the website feel more like a native app.
Source: https://en.wikipedia.org/wiki/Single-page_application
Razor components (.razor) can include page directives which will used by Blazor for page routing.
Routing is in Blazor the mechanism that matches classic URLs to Razor components/pages.
If a Razor component for example includes the following @page directive.
@page “/home”
Blazor will render this component and send it to the browser when the user enters the following URL in the browser.
https://<FQDN Web App>/home
Blazor also supports multiple routes for a component, so you can add two @page directives to the component and Blazor will render for both URLs the component and send it to the users browser.
@page “/home”
@page “/home2”
More about routing in Blazor you will find at the following website.
Blazor University – Routing
https://blazor-university.com/routing/
You will also find the _Host.cshtml file in the Pages folder which is the root page of the app implemented as a Razor page. When any page of the app is initially requested like for example the index.razor, this page is rendered and returned in the response.
In .NET 6 there is a _host.cshtml and _layout.cshtml where the _layout.cshtml is referenced in the _host.cshtml.
It seems that Microsoft changed this in .NET 7 back like before in .NET 3 and .NET 5 to just have a _Host.cshtml.
This page also loads all css and JavaScript files. Further it loads the _framework/blazor.server.js file which I described in Part I.
The Shared folder will include razor components without the @page directive. These are classic components which can be used by other components and mainly by components with the @page directive.
For example the navigation menu you will find here in form of a razor component NavMenu.razor which is included in the MainLayout.razor component which by itself is also homed in the Shared folder.
In an app created from a Blazor project template, the MainLayout component is the app’s default layout.
You can also use the @layout Razor directive to apply a layout to a routable Razor component that has an @page directive.
Directly in the root folder you will find the _Imports.razor, App.razor, appsettings.json and Program.cs file.
The App.razor file is the root component of the app that sets up client-side routing using the Router component. The Router component intercepts browser navigation and renders the page that matches the requested address.
The _Imports.razor file includes common Razor directives to include in the app’s components (.razor), such as @using directives for namespaces.
The appsettings.json file provides configuration settings for the app.
Finally the Program.cs file it the app’s entry point that sets up the ASP.NET Core host and contains the app’s startup logic, including service registrations and request processing pipeline configuration.
Source: https://learn.microsoft.com/en-us/aspnet/core/blazor/project-structure?view=aspnetcore-7.0
Start Debugging the web app
When you now start debugging the web app by pressing F5 you will see the following blazor app from the predefined default template.
I will use later this ready to use web app as foundation to build a complete new blazor web app with its own layout.
We will see this in the next upcoming posts and some nice features we can implement with Blazor.
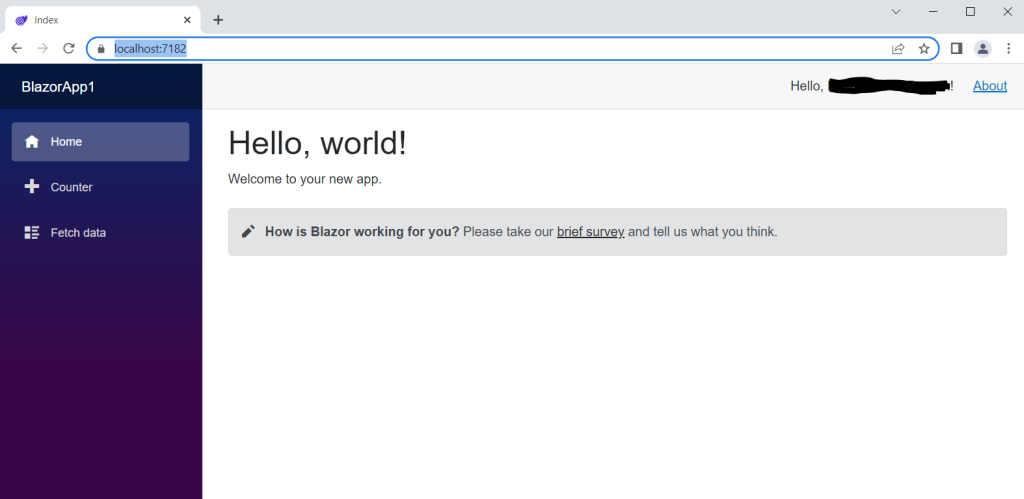
In order to understand the basics about Blazor, I would also strongly recommend to watch the 11 episodes from Jeff Fritz on YouTube or Microsoft directly. On YouTube you get a better navigation through the separate episodes.
Jeff Fritz will introduce you here to Blazor and really great explains the basics.
What is Blazor [1 of 11] | Blazor for Beginners
https://www.youtube.com/watch?v=9BhbGbTsyeE&list=PLdo4fOcmZ0oUJCA3DCzKT79Oe3kdKEceX&index=2Blazor Tutorial – Build your first Blazor app
https://dotnet.microsoft.com/en-us/learn/aspnet/blazor-tutorial/next
In Part III I will show how to use a custom layout template for your new Blazor web app.
Blazor Server Series
Blazor Server – Basics Part 1
https://blog.matrixpost.net/blazor-server-basics-part-i/Blazor Server – Basics Part 2
https://blog.matrixpost.net/blazor-server-basics-part-ii/Blazor Server – Basics Part 3 – Custom Layout
https://blog.matrixpost.net/blazor-server-basics-part-iii-custom-layout/Blazor Server – Basics Part 4 – Program.cs File
https://blog.matrixpost.net/blazor-server-basics-part-iv-program-cs-file/Blazor Server – Basics Part 5 – Authentication and Authorization
https://blog.matrixpost.net/blazor-server-basics-part-v-authentication-and-authorization/Blazor Server – Basics Part 6 – Query the on-premise Active Directory
https://blog.matrixpost.net/blazor-server-basics-part-vi-query-the-on-premise-active-directory/Blazor Server – Basics Part 7 – C# Events, Delegates and the EventCallback Class
https://blog.matrixpost.net/blazor-server-basics-part-vii-c-events-and-delegates/Blazor Server – Basics Part 8 – JavaScript interoperability (JS interop)
https://blog.matrixpost.net/blazor-server-basics-part-viii-javascript-interoperability-js-interop/Blazor Server – Basics Part 9 – Responsive Tags and Chips
https://blog.matrixpost.net/blazor-server-basics-part-ix-responsive-tags-and-chips/Blazor Server – Basics Part 10 – MS SQL Server Access and Data Binding
https://blog.matrixpost.net/blazor-server-basics-part-10-ms-sql-server-access-and-data-binding/Blazor Server – Basics Part 11 – Create a Native Blazor UI Toggle Switch Component
https://blog.matrixpost.net/blazor-server-basics-part-11-native-blazor-toggle-switch-by-using-the-eventcallback-class-and-css/Blazor Server – Basics Part 12 – Create a Native Blazor UI Toggle Button Component
https://blog.matrixpost.net/blazor-server-basics-part-12-create-a-native-blazor-ui-toggle-button-component/
Links
ASP.NET Core Blazor project structure
https://learn.microsoft.com/en-us/aspnet/core/blazor/project-structure?view=aspnetcore-7.0Blazor Tutorial – Build your first Blazor app
https://dotnet.microsoft.com/en-us/learn/aspnet/blazor-tutorial/introBlazor Tutorial – Build your first Blazor app –> Let Jeff walk you through building a full Blazor app from start to finish
https://dotnet.microsoft.com/en-us/learn/aspnet/blazor-tutorial/nextWhat is Blazor [1 of 11] | Blazor for Beginners
https://www.youtube.com/watch?v=9BhbGbTsyeE&list=PLdo4fOcmZ0oUJCA3DCzKT79Oe3kdKEceX&indexBlazor University – Routing
https://blazor-university.com/routing/